Java 8 introduced the Stream API, which revolutionized how we process collections. Mastering Stream API is crucial for any Java 8 Interview as it improves code efficiency, readability, and performance. This blog will cover the most frequently asked Stream API questions along with explanations and code examples.
What is JAVA 8 Stream API? How do create Stream, and Lambda expressions, what are the main terminal and intermediate functions of JAVA 8 Stream API. I recommend first going and reading about these topics.
Introduction to Java 8 Stream API
Java 8 introduced the Stream API, a powerful feature for processing collections efficiently. Unlike traditional loops, streams enable functional-style operations, making code more readable and optimized for performance. With methods like map()
, filter()
, reduce()
, and sorted()
, the Stream API allows parallel processing, lazy evaluation, and pipeline-based execution. Understanding Stream API is essential for any Java 8 interview, as it helps in writing concise and efficient code. In this guide, we’ll explore commonly asked Java 8 Stream API interview questions with real-world examples and explanations. 🚀
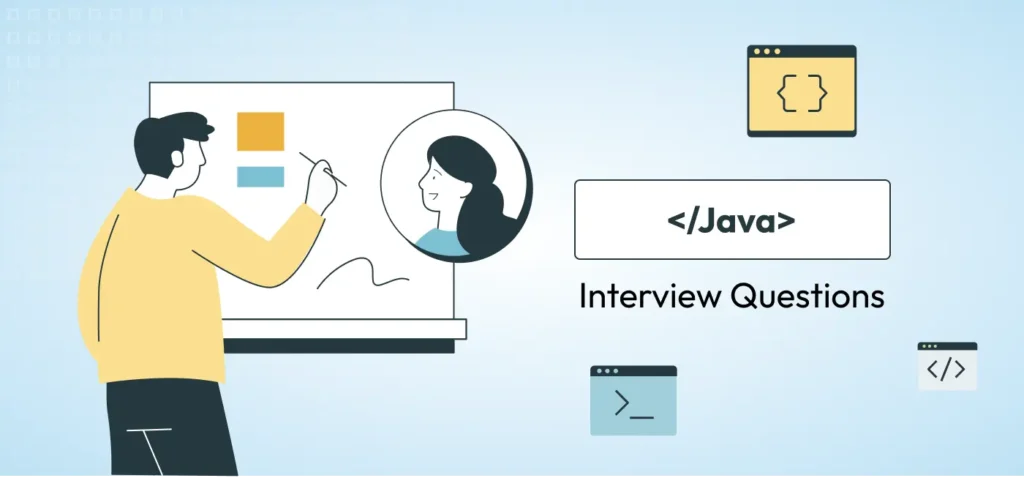
1. What is Stream API in Java 8?
Answer:
The Stream API is a feature in Java 8 that allows functional-style operations on collections of data. It enables developers to process large data efficiently using methods like map
, filter
, and reduce
.
Example:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class StreamExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("John", "Jane", "Jack", "Jill");
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("J"))
.collect(Collectors.toList());
System.out.println(filteredNames);
}
}
Output:
[John, Jane, Jack, Jill]
2. Difference Between Stream and Collection
Answer:
Feature | Collection | Stream |
---|---|---|
Storage | Stores elements | Doesn’t store elements |
Iteration | External iteration | Internal iteration |
Modification | Can modify elements | Can’t modify elements |
Usage | Can be reused | Can’t be reused |
3. How to Use map()
in Stream API?
Answer:
The map()
function is used for transforming elements of a collection.
Example:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class StreamMapExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
List<Integer> squaredNumbers = numbers.stream()
.map(n -> n * n)
.collect(Collectors.toList());
System.out.println(squaredNumbers);
}
}
Output:
[1, 4, 9, 16, 25]
4. How to Filter Elements in Stream API?
Answer:
The filter()
method is used to select elements based on a condition.
Example:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class StreamFilterExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(10, 15, 20, 25, 30);
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
System.out.println(evenNumbers);
}
}
Output:
[10, 20, 30]
5. What is reduce()
in Stream API?
Answer:
The reduce()
method is used for aggregation (sum, average, etc.).
Example:
import java.util.Arrays;
import java.util.List;
public class StreamReduceExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
int sum = numbers.stream()
.reduce(0, Integer::sum);
System.out.println(sum);
}
}
Output:
15
6. How to Sort Elements Using Stream API?
Answer:
The sorted()
method is used for sorting elements.
Example:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class StreamSortExample {
public static void main(String[] args) {
List<Integer> numbers = Arrays.asList(5, 3, 8, 1, 2);
List<Integer> sortedNumbers = numbers.stream()
.sorted()
.collect(Collectors.toList());
System.out.println(sortedNumbers);
}
}
Output:
[1, 2, 3, 5, 8]
7. What is flatMap()
in Java 8 Stream API?
Answer:
The flatMap()
function is used to flatten a stream of collections.
Example:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class StreamFlatMapExample {
public static void main(String[] args) {
List<List<String>> namesList = Arrays.asList(
Arrays.asList("John", "Jane"),
Arrays.asList("Jack", "Jill")
);
List<String> names = namesList.stream()
.flatMap(List::stream)
.collect(Collectors.toList());
System.out.println(names);
}
}
Output:
[John, Jane, Jack, Jill]
Conclusion
Mastering Stream API is crucial for cracking any Java 8 Interview. Understanding methods like map()
, filter()
, reduce()
, and flatMap()
helps in writing clean and efficient code. Keep practicing these concepts to ace your next Java 8 Interview!